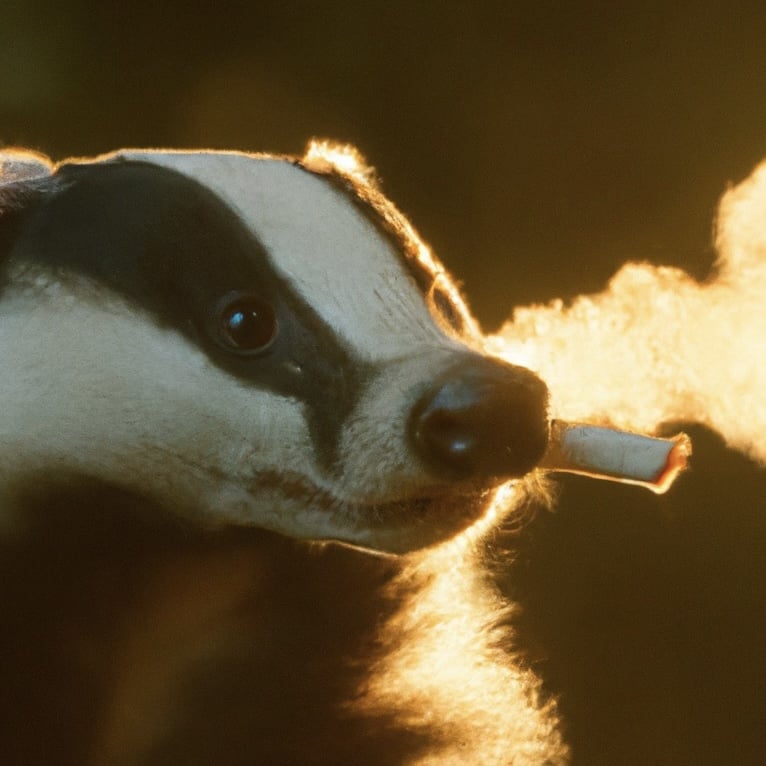
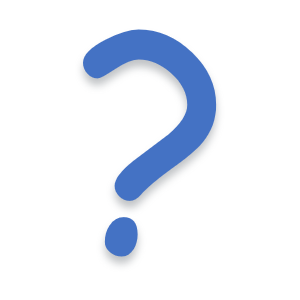
It’s not that bad, you might have a couple of moments where you suddenly second guess yourself, particularly on smaller country roads, or car parks. Just remember you the driver should be nearest to the center of the road and you’ll be fine. If you pre book you might have a chance of renting an automatic to make things even easier.
Maybe also look ip a good component library, for C# I use MudBlazor, which has great documentation that helps a lot to pick the right component for the job, and a lot of the hard work is already done in terms of styles/themes.
For my apps I use the MVVM pattern and write all the backend logic first, then that helps me narrow down the right way to display it.