
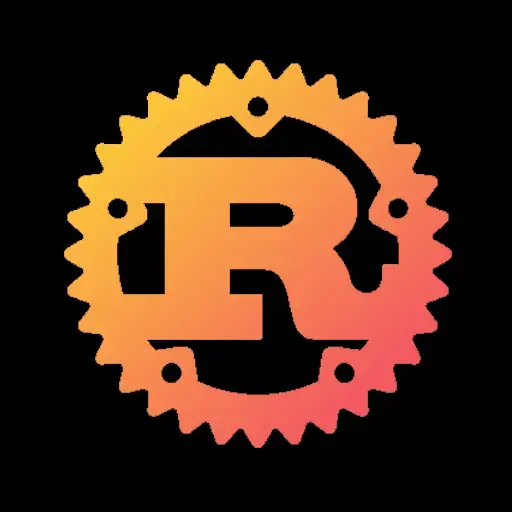
That sounds like fun! Wow. How stable is it at the moment?
That sounds like fun! Wow. How stable is it at the moment?
Working on some form of inheritance in rust. It’s my first foray into procedural macros and so far it’s fun. The idea is quite simple: generate structs with common attributes (and eventually functions) instead writing them yourself.
use inheriters::specialisations;
specialisations!(
struct Parent {
attr1: u8,
}
#[inherit(Child)]
struct Child {
attr2: u8,
}
);
becomes
struct Parent {
attr1: u8,
}
struct Child {
attr1: u8,
attr2: u8,
}
not
struct Parent {
attr1: u8,
}
struct Child {
attr1: u8,
parent: Parent,
}
The latter leads to indirection which I’m not a fan of.
Last week I squashed one bug on the order of attributes according to inheritance. In the example above attr2
was coming before attr1
. A feature is nearly done to exclude the Parent
from the output and only output the child. That’s useful for parents that just serve as holders for shared attributes.
The goal for v1 was to also support basic inheritance of implementations: Parent
has an impl
block, then that block is copied for the Child
. Not sure yet if I’ll implement overrides in v1 or v2. Overrides being if Parent
implements do_something()
and Child
does too, then the implementation of Parent
is not copied into the impl
block.
That’s what I’ll try to tackle in the coming weeks.
Great, thank you! I’ll be moving on to mkDerivation
soon. Hopefully budding packagers will be less intimidated than I was when I started.
Jottacloud is what I want to use. Unlimited storage for ~100€/month
Close behind is 1fichier for 2€/TB/month or 12€/TB/year, but they are in France and “uptobox” (a similar provider) was shutdown by the US on French soil because they allowed providing links to the files.
You can probably find others in the list of storage systems supported by rclone
This week some more work was done on inheriteRS to support inheriting non-trait implementations of functions.
Basically
use inheriters::specialisations; specialisations!( struct Parent { attr1: u8, } impl Parent { fn from_parent(self) -> u8 { 8 } fn overridden(self) -> u8 { self.attr1 } } #[inherit(Child)] struct Child { attr2: u8, } impl Child { fn overridden(self) -> u8 { self.attr2 } } );
results in
struct Parent { attr1: u8, } impl Parent { fn from_parent(self) -> u8 { 8 } fn overridden(self) -> u8 { self.attr1 } } struct Child { attr1: u8, // new attr2: u8, } impl Child { fn from_parent(self) -> u8 { 8 } // new fn overridden(self) -> u8 { self.attr2 } }
There might be some clean-up necessary code-wise and the README has to be expanded. But the project is well on its way to version 1! It’s a pity codeberg doesn’t have easy CI/CD yet nor domain hosting e.g inheriters.codeberg.io or something. So auto-formatting, testing, auto-tagging, etc. will have to come once I can convince myself to setup a VPS somewhere that hosts all that.