
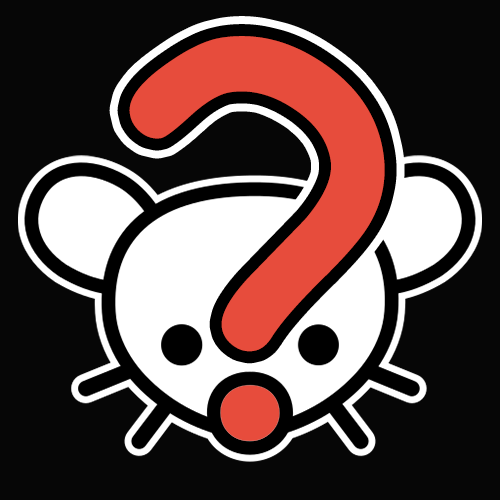
If the head needs to be empty, I find that droney Japanese noise is the best way to get that. Example: Aube - Flare
If the head needs to be empty, I find that droney Japanese noise is the best way to get that. Example: Aube - Flare
Lots of great sf/fantasy authors mentioned already, including some I’d argue for as great writers regardless of genre (Ursula K. Le Guin, Gene Wolfe, N. K. Jemisin).
I have three more to suggest in this genre and from this period:
C. J. Cherryh (Cyteen, Foreigner series, lots more) uses the lens of alien societies – just different enough from ours – to make us look critically at the structure of our own;
Sheri S. Tepper (Grass, Raising the Stones, The Gate to Women’s Country) carries one or another of the dark currents underlying our culture to its horrifying conclusion, and shows us what we get;
Lois McMaster Bujold (Vorkosigan saga) gives us a hilarious and improbable hero who utterly transcends his disabilities, in the end perfectly embodying what it seems he could never hope to be.
Was hoping someone would mention Shadow Hearts and Wild Arms! The PS2 truly was the janky AA JRPG console of all time. Also don’t forget
There are a few different issues interacting here.
The “family mode” users that require PIN are a child protection measure, and are not connected to Family Sharing. Remove the PIN from all adult accounts. Now you will see your whole library and be able to go to the store, and when you switch to your son’s user, he will not be able to go to the store and will only see the games you have done “Add to Family Games” on. This is how my library is set up: sharing to my partner and child, only child’s account has PIN.
I don’t know the cause of your experience with the keyboard, but if you remove the PIN from your own account, that should make it less painful.
This is just the way the Steam client works, not a Deck-specific feature: you are logged into one account until you change it. The PS5 is the same way.
In my experience, failure to separate game state between users is a game-by-game problem. Most Windows-native games running in Proton separate their saves by user correctly. (I do not know whether this happens because the Deck generates a completely clean Proton environment for each Steam user, or whether the Proton environment is shared and the game is just doing what it would do on a Windows PC to separate saves.) The games where I have seen saves wrongly shared, ironically, are all games with native Linux ports.
If you haven’t already, switch to your son’s account, unlock the PIN, and go through all the Steam multiplayer/chat settings. We have all that turned off for our child. As far as I know, a game family-shared to a user should behave exactly as if the user owned the game, from a functional point of view.
Have you tried the Ys series of action RPGs? Ys VIII is a lot of grindy monster whacking fun and runs great on Deck.
Baba Is You is fantastic, and I think its difficulty curve is much, much more reasonable in the beginning than Stephen’s Sausage Roll. I haven’t finished it, but I didn’t utterly bounce off it either.
Stephen’s Sausage Roll.
I play a lot of puzzle games. Some of them are pretty hard (the later levels of Tametsi take quite a while to crack).
But this one is on a completely different level. If there is a more brutally punishing sokoban-family game on existence, I have no idea what it might be.
Stephen, if he exists, is most likely condemned to roll sausages eternally in hell, for the sin of making this game.
Another vote for Cherryh - pretty much anything by Cherryh. And in the “journey” department, perhaps also look at John Varley’s Gaia trilogy (Titan, Wizard, Demon)? (Probably falls into your “excessive violence and some smut” category)
You might also try the “far future/dying Earth” genre as a way of getting the exploration without necessarily being tied to the space/hard sf milieu. I think the most awarded member of this subgenre (and I liked it quite a bit) is Gene Wolfe’s three Sun series (Book of the New Sun, Book of the Long Sun, Book of the Short Sun).
Old, but I think at least inspired or adjacent: Apparat Organ Quartet - Romantika
Swans - Song for Dead Time
So bury your trust beneath the ground with me, dear
And lay your loneliness down for the sun to burn
To sand…
Various groups are running fan servers for Monster Hunter Frontier since it shut down in 2019. There is a translated client that mostly works. Search for “rain frontier server” for more info.
Disclaimer: I haven’t tried any of this myself and I don’t know whether the client they distribute will give your computer encephalitis.
A few years ago Cook’s Illustrated published a recipe for turkey thigh confit. We figured, what the hell, let’s try it, if we aren’t going to do a ridiculous project like this at Thanksgiving, when will we?
It was incredible. Absolutely worth the work - the turkey comes out almost ham-like. We have done it every year since. It doesn’t scale to larger parties very well, but if you eat meat and have a small group (with 6 you won’t have leftovers), give it a try.
OK, that’s what I had kind of feared. Thank you!
Not like it will be such a hardship to finally finish it - just have to resist the temptation to play Monster Hunter instead :)
I enjoyed the first game very much but never finished it because I was distracted by some other shiny object. How much does 2 spoil the first game’s plot?
A few I’ve enjoyed that aren’t mentioned elsewhere so far:
Robin McKinley, The hero and the crown. If you’ve never read this, please, just go and do so, if you read nothing else on this entire response. The Newbery Medal it got was well deserved. (And it has princesses and dragons and wizards.)
Louise Cooper, Indigo (8 short books). Sealed ancient evil, cursed protagonist on heroic journey, talking animal companion. Just lots of fun all around.
Lois McMaster Bujold, The curse of Chalion series. Maybe a little more politics than you are looking for, but the divinity/magic system works well and I appreciate that the viewpoint characters are generally kind of old and busted. She is of course better known for the (excellent) Miles Vorkosigan military space opera series.
Sarah Monette and Elizabeth Bear, A companion to wolves et seq. Exactly what it says on the tin; the catch is that the viewpoint character of the first book becomes bonded to a female wolf, which radically changes how his culture sees him.
Elizabeth Moon, The deed of Paksenarrion. Basically what you’d get if you wrote down a really good D&D campaign (but mostly for only one viewpoint character). Formulaic in spots but enjoyable and well executed.
Other replies have mentioned Steven Brust’s Vlad Taltos books, which I enjoyed a lot; and David (and Leigh) Eddings, which were my first big-kid fantasy novels (as for many other other American children of the 70s and 80s). Another long series in something of the same vein as Eddings is Raymond E. Feist’s Riftwar saga; I haven’t read the entries after 2000, but before that it was a lot of fun.
Into Great Silence (originally: Die große Stille), a nearly wordless 3+ hour documentary about the monks of a Carthusian monastery in France.
You should watch it because it makes one really feel, as much as a movie can, their lives of meditative devotional repetition. I was able to touch for just a moment the peace they strive to immerse themselves in.
(I also felt cold. Those habits cannot possibly be enough in winter.)
This is all going to be “great gameplay” rather than “great story”.
If you have a way to play 3DS games and a tolerance for punishment in your JRPG dungeon crawlers, the Etrian Odyssey series has some of Yuzo Koshiro’s best work.
When you hit something more serious for the first time, you get this instead.
And, uh, there’s this memorable remix of the wandering-boss theme from the first game.
You already have Persona games in your list, but let’s add some more Shin Megami Tensei:
Finally … Monster Hunter is an incredibly fun and addictive game series, and though it doesn’t center the music quite as much as a typical JRPG does, it’s got some great stuff. I particularly like the soundtrack of MH: World – although the newer game MH: Rise tried something new with its mostly choral arrangements, I think they are not quite as memorable overall.
Arena battle from Monster Hunter: World (it’s particularly impressive the way this theme, the small-monsters arena battle theme, and several of the town themes reuse the same melodies in different arrangements)
Monster Hunter World is five years old and holds up great.
bask in the sun halfway up the Ancient Forest with a Tobi-Kadachi (giant white electric flying squirrelsnake; chill until you hit it)
climb up to the top of the Coral Highlands cat colony and watch the sky jellyfish float by in the sunset
share a hot spring with snow monkeys in the Hoarfrost Reach
They did a great job of making the maps feel like a living system that goes on while you’re not there. (Sadly, this is much less true of the newest MH game, Rise, where the maps are full of traversal puzzles but the wildlife pretty much all exists only to attack you.)
That is gorgeous! I do a lot of curry, tonkatsu, okonomiyaki, etc but I have never tried ramen - always thought the broth would be a huge project. But you make it look so good!
Put a shocking amount of time into Unicorn Overlord last week.
I think they executed the cross between Fire Emblem and Ogre Battle very well. Squad composition makes up for the lack of individual customization that is typical of the FE lineage of strategy RPGs (as opposed to the FFT/Tactics Ogre line). The overworld management is a fun exploration side activity that isn’t as time-consuming as Three Houses’s social stuff. Basiscape brought its usual excellent soundtrack, and Vanillaware their usual impressively detailed art. Plot is whatever, I don’t play these games for the plot, I play them to make anime sprites stab each other so numbers go up. So, yeah, it’s fun.
(No, I don’t actually like Disgaea that much, mostly because “figuring out how to break the game is the game” doesn’t appeal to me.)