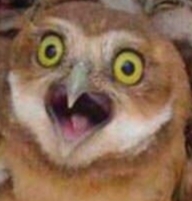
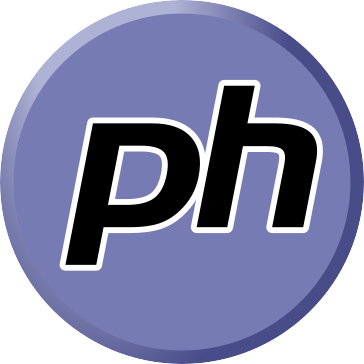
I’m not missing your points, even as you change them. I’ve agreed that JS sucks. I’ve agreed that errors can be more helpful. I’m not trying to argue with you about that. What I have said, from the beginning, is that in the code you originally presented a behavioural change for setMonth
will not help you find the problem any faster. Test failures for the wrong output occur just as often as test failures for errors, on exactly the same few days each year. The API change gives no advantage for the specific function this discussion started with in this regard. However, an approach that avoids inconsistency will, because in this particular instance, that is the real source of the problem. That is all.
In that context—the one you started with—it does not matter that there is often good reason to call Date()
without arguments. The getMonthName
function presented, effectively an array lookup, should produce the same output for any given input every time. It has no reason to engage in any behaviour that varies from day to day.
There is absolutely nothing wrong with getting the current date.
Bluntly, the code you presented fails precisely because it gets the current date where it should create a more specific one, and then fails to deal with that variation appropriately. You can keep distracting yourself with language design decisions, but that won’t help you avoid this particular type of problem in the future because that’s not where it is.
Getting the current date is often fine. In this specific instance, it is not. That is why the function doesn’t work. If you are missing that point, as much as I appreciate your enthusiasm in continuing the conversation, I will take the L (and the code that actually works) and move on.
But that isn’t what it does. From the original post:
That is a function which is meant to take a number (presumably 1 to 12) and return a localized name for it. This is essentially an array lookup and should return the same output for a given input (and locale) every time it is called. If the intent is to return a value relative to the current date, it is even more wrong, since it should gather the month from the current date, not the function paramenter. This claim of intent, not present in the original post, is an example of you changing your story over time.
No, it wouldn’t. As I have said before, testing for unexpected return values is just as effective as testing for errors, that is, not very with the function originally presented under sensible assumptions. If the function actually did look like the intent you claim, the tests would be different, necessarily replacing
Date
for consistent runs, but would be equally likely to catch the problem whether failing on value or error. And if you are eschewing testing and relying on runtime crashes, you have bigger problems.Given that I have agreed and commiserated, and neither of us can change JavaScript, there is nothing to be gained from pursuing this complaint. In contrast, what I have tried to say, if followed, would give you an approach that leads to more reliable code, even in the face of undesirable APIs.
I had thought that worth pursuing, and had thought you worth investing my considerable time. Alas, I can only lead you to the water…